To Override a Virtual Method
- You can define the override method with exactly the same signature—that is, the return value, the name of the method, and all method parameters—as the overridden base virtual method has. As a result, the method is added to the queue of all override methods. When the system invokes the base method, all methods in the queue are executed sequentially, from the first one to the last one. The lower the level of the graph extension, the earlier the system invokes the override method.
- You can define the override method with an additional parameter, which represents the delegate for one of the following:
- The override method with an additional parameter from the extension of the previous level, if such a method exists
- The base virtual method, if no override methods with additional parameters declared within lower-level extensions exist
Override Method That Is Added to the Override Method Queue
By declaring an override method with exactly the same signature as the overridden base virtual method has, you extend the base method execution. The base BLC method is replaced at run time with the queue of methods, which starts with the base BLC method. When the system invokes the base method, all methods in the queue are executed sequentially, from the first one to the last one. The lower the level the BLC extension has, the earlier the system invokes the override method. If the system has invoked the base method, you have no option to prevent the override method queue from execution. To prevent the base method executions, see the Override Method That Replaces the Original Method section below.
- Create the graph extension, as described in To Start the Customization of a Graph.
- In the Code Editor, click View Source to view the code of the base
graph in the Source Code browser.Note:In an instance of MYOB Acumatica, a repository with the original C# source code of the application is kept in the \App_Data\CodeRepository folder of the website.
- In the browser, select and copy the code of the method.
- In the Code Editor, paste the method code in the graph extension.
- In the graph extension, insert the PXOverride attribute immediately before the method signature.
- Clear the method body.
- Develop the code of the method.
- Click Save in Code Editor to save your changes.
Override Method That Replaces the Original Method
The override method with an additional parameter replaces the base BLC virtual method. When the virtual method is invoked, the system invokes the override method with an additional parameter of the highest-level BLC extension. The system passes a link to the override method with an additional parameter from the extension of the previous level, if such a method exists, or to the base virtual method.
public class BaseBLCExt : PXGraphExtension<BaseBLC>
{
public delegate ReturnType MyMethodDelegate([List of Parameters]);
[PXOverride]
public ReturnType MyMethod([List of Parameters,] MyMethodDelegate baseMethod)
{
return baseMethod(adapter);
}
}
You can decide whether to call the method pointed to by the delegate. By invoking the base method, you also start the execution of the override method queue.
- Create the graph extension, as described in To Start the Customization of a Graph.
- In the Code Editor, click Override Method, as shown in the screenshot below.
- In the Selected column of the Select Methods to Override dialog box, which opens, select the method to be overridden.
- Click Save to add the code template for the override method to
the graph extension.
Figure 1. Selecting a method to be overridden
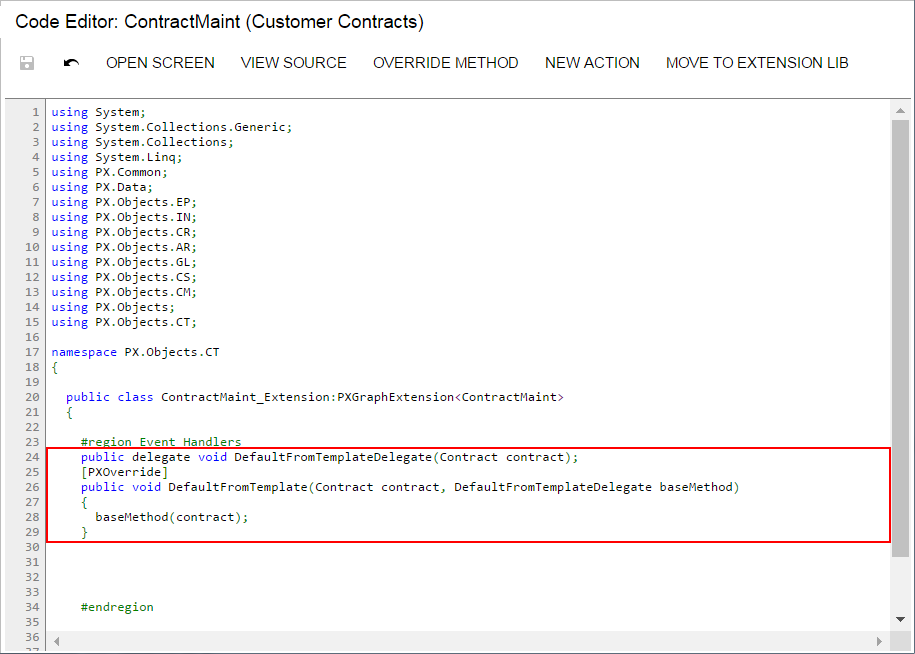
When you publish the customization project, the platform saves the graph extension as a C# source code file in the App_RuntimeCode folder of the website. You can later develop the event handler in Microsoft Visual Studio. (See Integrating the Project Editor with Microsoft Visual Studio for details.)