Graph Extensions
This topic explores the ways provided by the MYOB Acumatica Customization Platform to define business logic controller (BLC, also referred as graph) extensions of different levels, and shows how different BLC extensions can interact.
To declare an extension of a BLC, you derive a class from
PXGraphExtension<T>
.
First-Level BLC Extension
The example below shows a declaration of a first-level BLC extension.
class BaseBLCExtension : PXGraphExtension<BaseBLC>
{
public void SomeMethod()
{
BaseBLC baseBLC = Base;
}
}
The extension class includes the read-only Base property, which returns an instance of the base BLC.
Second-Level BLC Extension
The example below shows a declaration of a second-level BLC extension.
class BaseBLCExtensionOnExtension :
PXGraphExtension<BaseBLCExtension, BaseBLC>
{
public void SomeMethod()
{
BaseBLC baseBLC = Base;
BaseBLCExtension ext = Base1;
}
}
The extension class includes the following:
- The read-only Base property, which returns the instance of the base BLC
- The read-only Base1 property, which returns the instance of the first-level BLC extension
Application of a Graph Extension to Graph Inheritors
The graph extension applies not only to a graph specified in the graph extension’s declaration but to all graphs derived from the graph specified in the graph extension’s declaration.
Suppose, you have a base graph named ARInvoiceEntry, two graphs named SOInvoiceEntry and ARSpecificInvoiceEntry which are derived from ARInvoiceEntry and a graph extension named ARInvoiceEntryAdvanceTaxesExt declared for ARInvoiceEntry as shown in the following diagram.
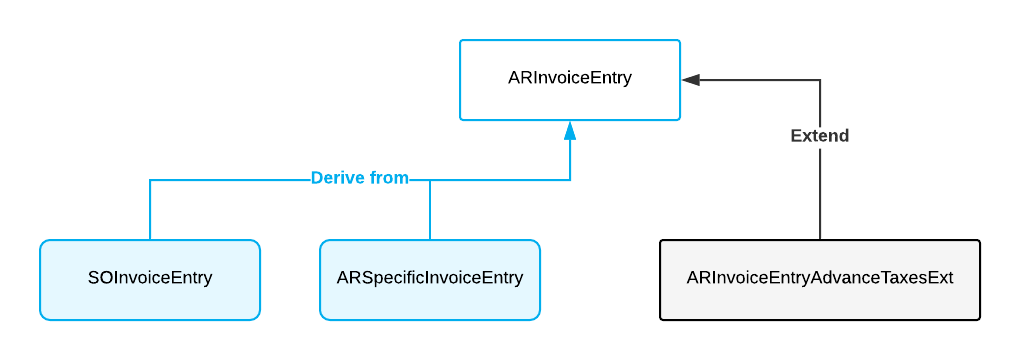
MYOB Acumatica Framework applies members declared in the ARInvoiceEntryAdvanceTaxesExt to all graphs: ARInvoiceEntry, ARSpecificInvoiceEntry, and SOInvoiceEntry.
Two Variants of a Higher-Level BLC Extension
A definition of a higher-level BLC extension has two possible variants. In the first variant, you derive the extension class from the PXGraphExtension generic class with two type parameters, where the first type parameter is set to an extension of the previous level. In the second variant, you derive the extension class from the PXGraphExtension generic class with the same number of type parameters as the level of the extension of the new class. In this case, you set type parameters to extension classes from all lower extension levels, from the previous level down to the base class.
First Variant of a Higher-Level BLC Extension
The example below shows a declaration of a third- or higher-level BLC extension that is derived from the PXGraphExtension generic class with two type parameters.
class BaseBLCMultiExtensionOnExtension :
PXGraphExtension<BaseBLCExtensionOnExtension, BaseBLC>
{
public void SomeMethod()
{
BaseBLC BLC = Base;
BaseBLCExtensionOnExtension prevExt = Base1;
}
}
An extension class defined in this way includes the following:
- The read-only Base property, which returns the instance of the base BLC
- The read-only Base1 property, which returns the instance of the BLC extension from the previous level
Second Variant of a Higher-Level BLC Extension
The example below shows a declaration of a third- or higher-level BLC extension that is derived from the PXGraphExtension generic class with three or more type parameters.
class BaseBLCAdvMultiExtensionOnExtension :
PXGraphExtension<BaseBLCExtensionOnExtension, BaseBLCExtension, BaseBLC>
{
public void SomeMethod()
{
BaseBLC BLC = Base;
BaseBLCExtension ext = Base1;
BaseBLCExtensionOnExtension extOnExt = Base2;
}
}
An extension class defined in this way includes the following:
- The read-only Base property, which returns the instance of the base BLC
- The read-only BaseN properties for all extension levels below the current level, where N is the sequence number of an extension level
Conceptually, a BLC extension is a substitution of the base BLC. The base BLC is replaced at run time with the merged result of the base BLC and every extension the platform found. The higher level of a declaration an extension has, the higher priority it obtains in the merge operation.