Processing Form: To Create the UI of a Processing Form
The following activity will walk you through the process of developing the UI of a processing form.
Story
Suppose that you need to develop the Assign Work Orders (RS501000) form in the Modern UI. The form will have the Selection area and a table, as shown in the following screenshot.
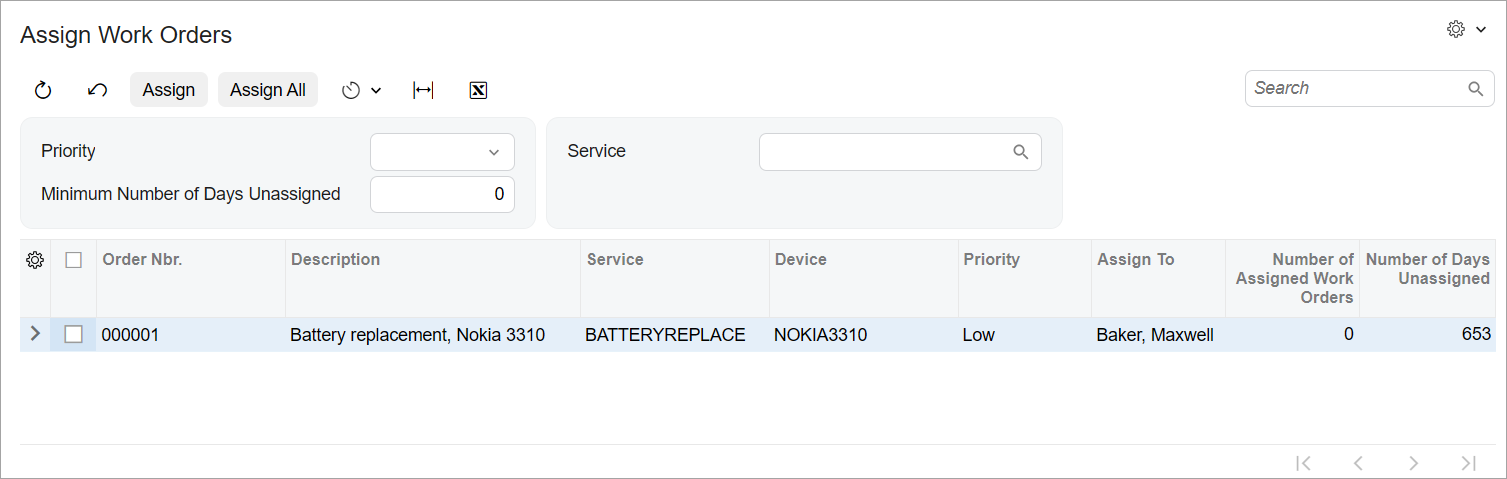
You have already implemented the backend for the form, which includes the
RSSVAssignProcess
graph and the RSSVWorkOrder
and RSSVWorkOrderToAssignFilter
data access classes (DACs). You
have also already added the corresponding tables to the application database.
Process Overview
You will create TypeScript and HTML files for the Assign Work Orders (RS501000) form. In the TypeScript file, you will define the screen class and view classes for the form. You will also define the following views:
Filter
, which is bound to the Selection area of the formWorkOrders
, which is bound to the table of the form
In the HTML file, you will define the layout of the form.
System Preparation
Before you begin creating the UI of the Assign Work Orders (RS501000) form, do the following:
- Complete the following prerequisite activity: Modern UI Development: To Deploy an Instance with Custom Forms and the Modern UI. Make sure the
prepared instance contains the following items:
- The
RSSVAssignProcess
graph in the customization code - The
RSSVWorkOrder
andRSSVWorkOrderToAssignFilter
DACs in the customization code - The
RSSVWorkOrder
database table
- The
- To take the prerequisite actions and build the source code for the first time, perform the following prerequisite activity: Modern UI Development: To Build the Source Code of All MYOB Acumatica Forms for Modern UI Development.
Step 1: Creating Files for the Form
To implement the Modern UI version of the Assign Work Orders (RS501000) form, you need to create the TypeScript and HTML files for the form. Create the files as follows:
- In the FrontendSources\screen\src\screens folder of your MYOB Acumatica instance, create a folder with the RS name if one has not been created yet. You will store the UI sources for all forms with the RS prefix in this folder.
- In the FrontendSources\screen\src\screens\RS folder, create a folder with the RS501000 name if it has not been created yet.
- In the FrontendSources\screen\src\screens\RS\RS501000
folder, create the following files:
- RS501000.ts
- RS501000.html
Step 2: Defining the Screen Class in TypeScript
To define the view of the Assign Work Orders (RS501000) form in the TypeScript file of the form, you define a screen class and a property for the data view of the form. Do the following:
- In the RS501000.ts file, add the import
directives as
follows.
import { PXScreen, createCollection, graphInfo, viewInfo, createSingle, } from "client-controls";
- Define the screen class for the form, as the following code shows. The class
name is the ID of the
form.
export class RS501000 extends PXScreen {}
- For the screen class, add the graphInfo decorator, and
specify the graph and the primary view of the form in the decorator properties,
as the following code
shows.
@graphInfo({ graphType: "PhoneRepairShop.RSSVAssignProcess", primaryView: "Filter" }) export class RS501000 extends PXScreen {}
- Define the property for the data views of the form, as the following code shows.
To initialize the data view of the Selection area of the form, you should use
the createSingle method. For the data view that is used to
display a table, you need to initialize the property with the
createCollection method. The method takes as the input
parameter an instance of the view class, which you will define in the next
step.Attention:The names of the data view properties should be the same as those in the graph. For example, if the
WorkOrders
view is declared in theRSSVAssignProcess
graph, the property with the same name should be declared in theRS501000
screen class.export class RS501000 extends PXScreen { @viewInfo({containerName: "Filter Parameters"}) Filter = createSingle(RSSVWorkOrderToAssignFilter); @viewInfo({containerName: "Work Orders to Assign"}) WorkOrders = createCollection(RSSVWorkOrder); }
In the viewInfo decorators, you have specified the name of the container for the Selection area and the table.
Step 3: Defining the View Class for the Selection Area in TypeScript
In the TypeScript file of the form, you need to define a view class for the primary
data view of the Assign Work Orders (RS501000) form, which is
Filter
.
Proceed as follows:
- In the RS501000.ts file, update the list of import
directives, as the following code
shows.
import { PXScreen, createCollection, graphInfo, viewInfo, createSingle, PXView, PXFieldOptions, PXFieldState, } from "client-controls";
- Define the
RSSVWorkOrderToAssignFilter
class as follows.export class RSSVWorkOrderToAssignFilter extends PXView {}
- In the view class, specify the properties for all data fields of the data view
that should be displayed in the UI, as shown below. You use the name of the data
field as the property
name.
export class RSSVWorkOrderToAssignFilter extends PXView { Priority: PXFieldState<PXFieldOptions.CommitChanges>; TimeWithoutAction: PXFieldState<PXFieldOptions.CommitChanges>; ServiceID: PXFieldState<PXFieldOptions.CommitChanges>; }
All fields of the view should be defined so that changes are committed to the server; therefore, you have used the PXFieldOptions.CommitChanges option for the property type.
Step 4: Defining the View Class for the Table in TypeScript
In the TypeScript file of the form, you need to define a view class for the table on
the Assign Work Orders (RS501000) form, which is RSSVWorkOrder
.
Proceed as follows:
- In the RS501000.ts file, add gridConfig, columnConfig, and GridPreset to the list of import directives.
- Define the
RSSVWorkOrder
view class as follows.export class RSSVWorkOrder extends PXView { @columnConfig({ allowCheckAll: true }) Selected: PXFieldState; @columnConfig({ hideViewLink: true }) OrderNbr: PXFieldState; Description: PXFieldState; @columnConfig({ hideViewLink: true }) ServiceID: PXFieldState; @columnConfig({ hideViewLink: true }) DeviceID: PXFieldState; Priority: PXFieldState; @columnConfig({ hideViewLink: true}) AssignTo: PXFieldState<PXFieldOptions.CommitChanges>; NbrOfAssignedOrders: PXFieldState; TimeWithoutAction: PXFieldState; }
For the
Selected
field, in the columnConfig decorator, you have specified that a user should be able to select all records on the page by clicking the check box in the column header.For the
OrderNbr
,ServiceID
,DeviceID
fields, andAssignTo
fields, in the columnConfig decorator, you have specified that the selector link should not be displayed.For the
AssignTo
field, you have specified that changes in this field should be committed to the server. - Add the grigConfig decorator to the
RSSVWorkOrder
view class, as the following code shows. In the grigConfig decorator, you must specify the preset property. Because the table is used on the processing form, you use the Processing preset. For details about presets, see Form Layout: Grid Presets.@gridConfig({ preset: GridPreset.Processing, autoAdjustColumns: true }) export class RSSVWorkOrder extends PXView { ... }
In the gridConfig decorator, you have also specified that the table width should be adjusted to the screen width. Otherwise, some of the columns may not be wide enough to display values.
- Save your changes.
Step 5: Defining the Layout in HTML
The Assign Work Orders (RS501000) form contains the Selection area and a table below it. The Selection area has two columns, which you can arrange by using the 17-17-14 template, which is a recommended one for processing forms. To define the layout of the form, do the following:
- Define the Selection area of the form by adding the
qp-template tag with the 17-17-14 template. For the first
two slots, define a fieldset, as shown in the following code. To leave the third
slot empty, do not specify any tags for
it.
<template> <qp-template id="form-Filter" name="17-17-14" class="equal-height" > <qp-fieldset id="fsColumnA-Filter" slot="A" view.bind="Filter" class="label-size-xm" > </qp-fieldset> <qp-fieldset id="fsColumnB-Filter" slot="B" view.bind="Filter"> </qp-fieldset> </qp-template> </template>
Each fieldset has been bound to the same
Filter
view.For details about the qp-template tag and slots, see Form Layout: Predefined Templates.
- In each fieldset, add the field tags for the fields that
should be displayed in the corresponding fieldset, as the following code
shows.
<qp-fieldset id="fsColumnA-Filter" slot="A" view.bind="Filter" class="label-size-xm" > <field name="Priority"></field> <field name="TimeWithoutAction"></field> </qp-fieldset> <qp-fieldset id="fsColumnB-Filter" slot="B" view.bind="Filter"> <field name="ServiceID"></field> </qp-fieldset>
In the first fieldset, you have also specified the width of the labels by using the label-size-xm class. For more details about CSS classes, see Form Layout: CSS Classes.
- Define the table that displays repair work orders: After the
qp-template tag, add the qp-grid tag
and bind it to the
WorkOrders
view, as the following code shows.<qp-grid id="grid-WorkOrders" view.bind="WorkOrders"> </qp-grid>
- Save your changes.
Step 6: Building and Viewing the Form
To build the source files for the Assign Work Orders (RS501000) processing form and view its Modern UI version, do the following:
- Run the following command in the FrontendSources\screen
folder of your
instance.
npm run build-dev -- --env screenIds=RS501000
- After the source files have been built successfully, launch your MYOB Acumatica instance, and open the Assign Work Orders form.
- On the form title bar, click Modern UI version of the Assign Work Orders form is displayed. The form should look similar to the form shown in the screenshot in the Story section of this activity. . The
- In the Priority box, select High. Make sure that only repair work orders with the High priority (if any) are displayed in the table.
- Click Cancel on the form toolbar.
- On the form toolbar, click Assign All. After all repair work orders in the table have been processed, a report opens with the result of processing.