Setup Form: A Form with Tabs
The following topic describes how to configure a form that consists of multiple tabs. (In the Classic UI, these forms were based on the TabView template.) The following screenshot shows an example of a form with this layout.
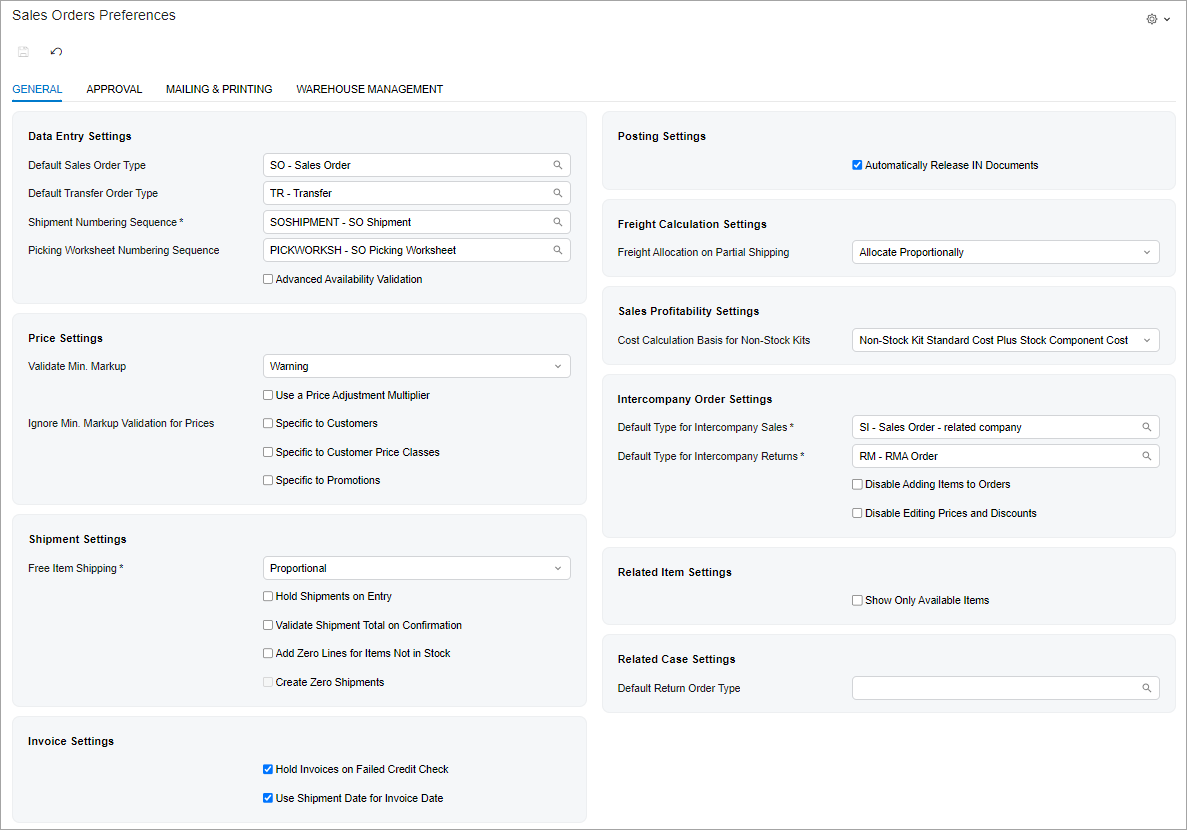
View Definition in TypeScript
To configure a setup form with multiple tabs in the TypeScript file, do the following:
- For the views that display one record, use the
createSingle
method. - For the views that display a grid, use the
createCollection
method. - For each view for a tab, use a class that extends
PXView
with the full list of fields to be displayed. - For each grid and its columns, use the
gridConfig
andcolumnConfig
decorators. For details, see Table (Grid).
The following code shows an example of the TypeScript configuration for the form in the screenshot above.
import {
createCollection,
createSingle,
PXScreen,
graphInfo,
viewInfo,
PXView,
PXFieldState,
PXFieldOptions,
columnConfig,
localizable,
gridConfig,
ScreenUpdateParams
} from "client-controls";
@graphInfo({ graphType: 'PX.Objects.SO.SOSetupMaint', primaryView: 'sosetup' })
export class SO101000 extends PXScreen {
@viewInfo({ containerName: "SO Preferences" })
sosetup = createSingle(sosetup);
@viewInfo({ containerName: "Approval" })
SetupApproval = createCollection(SetupApproval);
@viewInfo({ containerName: "Default Sources" })
Notifications = createCollection(Notifications);
@viewInfo({ containerName: "Default Recipients" })
Recipients = createCollection(Recipients);
@viewInfo({ containerName: "Warehouse Management" })
PickPackShipSetup = createSingle(PickPackShipSetup);
}
export class sosetup extends PXView {
DefaultOrderType: PXFieldState;
TransferOrderType: PXFieldState;
ShipmentNumberingID: PXFieldState;
PickingWorksheetNumberingID: PXFieldState;
AdvancedAvailCheck: PXFieldState;
MinGrossProfitValidation: PXFieldState;
UsePriceAdjustmentMultiplier: PXFieldState;
IgnoreMinGrossProfitCustomerPrice: PXFieldState;
IgnoreMinGrossProfitCustomerPriceClass: PXFieldState;
IgnoreMinGrossProfitPromotionalPrice: PXFieldState;
FreightAllocation: PXFieldState;
FreeItemShipping: PXFieldState;
HoldShipments: PXFieldState;
RequireShipmentTotal: PXFieldState;
AddAllToShipment: PXFieldState<PXFieldOptions.CommitChanges>;
CreateZeroShipments: PXFieldState<PXFieldOptions.CommitChanges>;
CreditCheckError: PXFieldState;
UseShipDateForInvoiceDate: PXFieldState;
AutoReleaseIN: PXFieldState;
SalesProfitabilityForNSKits: PXFieldState;
DfltIntercompanyOrderType: PXFieldState;
OrderType: PXFieldState;
DfltIntercompanyRMAType: PXFieldState;
DisableAddingItemsForIntercompany: PXFieldState;
DisableEditingPricesDiscountsForIntercompany: PXFieldState;
ShowOnlyAvailableRelatedItems: PXFieldState;
DefaultReturnOrderType: PXFieldState;
OrderRequestApproval: PXFieldState<PXFieldOptions.CommitChanges>;
}
export class SetupApproval extends PXView {
@columnConfig({ hideViewLink: true }) OrderType: PXFieldState;
AssignmentMapID: PXFieldState;
AssignmentNotificationID: PXFieldState;
}
@gridConfig({
preset: GridPreset.Details,
autoRepaint: ["Recipients"] })
})
export class Notifications extends PXView {
Active: PXFieldState<PXFieldOptions.CommitChanges>;
NotificationCD: PXFieldState;
@columnConfig({ hideViewLink: true }) NBranchID: PXFieldState;
@columnConfig({ hideViewLink: true }) EMailAccountID: PXFieldState;
@columnConfig({ hideViewLink: true }) DefaultPrinterID: PXFieldState;
@columnConfig({ hideViewLink: true }) ReportID: PXFieldState;
NotificationID: PXFieldState;
@columnConfig({ hideViewLink: true }) ShipVia: PXFieldState;
Format: PXFieldState;
RecipientsBehavior: PXFieldState;
}
@gridConfig({
preset: GridPreset.Details
})
export class Recipients extends PXView {
Active: PXFieldState;
ContactType: PXFieldState<PXFieldOptions.CommitChanges>;
OriginalContactID: PXFieldState;
ContactID: PXFieldState<PXFieldOptions.CommitChanges>;
Format: PXFieldState;
AddTo: PXFieldState;
}
export class PickPackShipSetup extends PXView {
ShowPickTab: PXFieldState<PXFieldOptions.CommitChanges>;
ShowPackTab: PXFieldState<PXFieldOptions.CommitChanges>;
ShowShipTab: PXFieldState<PXFieldOptions.CommitChanges>;
ShowReturningTab: PXFieldState<PXFieldOptions.CommitChanges>;
ShowScanLogTab: PXFieldState;
ShortShipmentConfirmation: PXFieldState;
ShipmentLocationOrdering: PXFieldState;
UseDefaultQty: PXFieldState;
ExplicitLineConfirmation: PXFieldState;
UseCartsForPick: PXFieldState;
DefaultLocationFromShipment: PXFieldState;
DefaultLotSerialFromShipment: PXFieldState;
EnterSizeForPackages: PXFieldState;
PrintShipmentConfirmation: PXFieldState;
PrintShipmentLabels: PXFieldState;
PrintCommercialInvoices: PXFieldState;
ConfirmEachPackageWeight: PXFieldState;
RequestLocationForEachItem: PXFieldState;
ConfirmToteForEachItem: PXFieldState;
AllowMultipleTotesPerShipment: PXFieldState;
PrintPickListsAndPackSlipsTogether: PXFieldState;
}
Layout in HTML
You define the layout of a setup form with tabs by adding the following tags to the HTML code of the form:
- A qp-tabbar tag with a nested qp-tab element for each tab. For qp-tabbar, you specify the ID of the tab that is displayed by default in active-tab-id.
- For each tab that does not contain a grid, a nested qp-template tag. For details on using templates, see Form Layout: Predefined Templates.
The following code shows the HTML template for the form in the screenshot above.
<template>
<qp-tabbar active-tab-id="tabGeneral" id="mainTabbar">
<qp-tab id="sosetup_tab" wg-container caption="General">
<qp-template name="17-17-14">
<div slot="A" class="v-stack">
<qp-fieldset id="pnlDataEntrySettings" view.bind="sosetup" caption="Data Entry Settings">
<field name="DefaultOrderType"></field>
<field name="TransferOrderType"></field>
<field name="ShipmentNumberingID"></field>
<field name="PickingWorksheetNumberingID"></field>
<field name="AdvancedAvailCheck"></field>
</qp-fieldset>
<qp-fieldset id="pnlPriceSettings" view.bind="sosetup" caption="Price Settings">
<field name="MinGrossProfitValidation"></field>
<field name="UsePriceAdjustmentMultiplier"></field>
<field name="IgnoreMinGrossProfitOptionsLabel" unbound replace-content>
<qp-label caption="Ignore Min. Markup Validation for Prices Specific To"></qp-label>
</field>
<field name="IgnoreMinGrossProfitCustomerPrice">
</field>
<field name="IgnoreMinGrossProfitCustomerPriceClass"></field>
<field name="IgnoreMinGrossProfitPromotionalPrice"></field>
</qp-fieldset>
<qp-fieldset id="pnlFreightCalc" view.bind="sosetup" caption="Freight Calculation Settings">
<field name="FreightAllocation"></field>
</qp-fieldset>
<qp-fieldset id="pnlShipmentSettings" view.bind="sosetup" caption="Shipment Settings">
<field name="FreeItemShipping"></field>
<field name="HoldShipments"></field>
<field name="RequireShipmentTotal"></field>
<field name="AddAllToShipment"></field>
<field name="CreateZeroShipments"></field>
</qp-fieldset>
<qp-fieldset id="pnlInvoiceSettings" view.bind="sosetup" caption="Invoice Settings">
<field name="CreditCheckError"></field>
<field name="UseShipDateForInvoiceDate"></field>
</qp-fieldset>
</div>
<div slot="B" class="v-stack">
<qp-fieldset id="pnlPostingSettings" view.bind="sosetup" caption="Posting Settings">
<field name="AutoReleaseIN"></field>
</qp-fieldset>
<qp-fieldset id="pnlSalesProfitability" view.bind="sosetup" caption="Sales Profitability Settings">
<field name="SalesProfitabilityForNSKits"></field>
</qp-fieldset>
<qp-fieldset id="pnlIntercompany" view.bind="sosetup" caption="Intercompany Order Settings">
<field name="DfltIntercompanyOrderType"></field>
<field name="DfltIntercompanyRMAType"></field>
<field name="DisableAddingItemsForIntercompany"></field>
<field name="DisableEditingPricesDiscountsForIntercompany"></field>
</qp-fieldset>
<qp-fieldset id="pnlRelatedItemSettings" view.bind="sosetup" caption="Related Item Settings">
<field name="ShowOnlyAvailableRelatedItems"></field>
</qp-fieldset>
<qp-fieldset id="pnlRelatedCaseSettings" view.bind="sosetup" caption="Related Case Settings">
<field name="DefaultReturnOrderType"></field>
</qp-fieldset>
</div>
</qp-template>
</qp-tab>
<qp-tab id="tabApproval" caption="Approval">
<div class="v-stack">
<div id="PXPanel1" wg-container>
<qp-fieldset id="groupRequireApproval" view.bind="sosetup">
<field name="OrderRequestApproval"></field>
</qp-fieldset>
</div>
<div>
<qp-grid id="gridApproval" view.bind="SetupApproval">
</qp-grid>
</div>
</div>
</qp-tab>
<qp-tab id="tabMailingAndPrinting" caption="Mailing & Printing">
<div id="formMailingAndPrinting" class="v-stack">
<qp-grid id="gridNS" view.bind="Notifications">
</qp-grid>
<qp-grid id="gridNR" view.bind="Recipients">
</qp-grid>
</div>
</qp-tab>
<qp-tab id="tabWarehouseManagement" caption="Warehouse Management">
<qp-template name="1-1-1">
<div slot="A" id="PickPackShipSetup_formScanSetup" wg-container class="v-stack">
<qp-label caption="These settings are specific to the current branch."></qp-label>
<qp-fieldset id="groupFulfillmentWorkflow" view.bind="PickPackShipSetup"
caption="Fulfillment Workflow">
<field name="ShowPickTab"></field>
<field name="ShowPackTab"></field>
<field name="ShowShipTab"></field>
<field name="ShowReturningTab"></field>
<field name="ShowScanLogTab"></field>
</qp-fieldset>
<qp-fieldset id="groupFulfillmentSettings" view.bind="PickPackShipSetup"
caption="Fulfillment Settings">
<field name="ShortShipmentConfirmation"></field>
<field name="ShipmentLocationOrdering"></field>
<field name="UseDefaultQty"></field>
<field name="ExplicitLineConfirmation"></field>
<field name="UseCartsForPick"></field>
<field name="DefaultLocationFromShipment"></field>
<field name="DefaultLotSerialFromShipment"></field>
<field name="EnterSizeForPackages"></field>
<field name="PrintShipmentConfirmation"></field>
<field name="PrintShipmentLabels"></field>
<field name="PrintCommercialInvoices"></field>
<field name="ConfirmEachPackageWeight"></field>
<field name="RequestLocationForEachItem"></field>
<field name="ConfirmToteForEachItem"></field>
<field name="AllowMultipleTotesPerShipment"></field>
<field name="PrintPickListsAndPackSlipsTogether"></field>
</qp-fieldset>
</div>
</qp-template>
</qp-tab>
</qp-tabbar>
</template>