Dialog Box: Configuration
A dialog box can contain a Summary area, a grid, and a footer with buttons, as shown in the following screenshot.
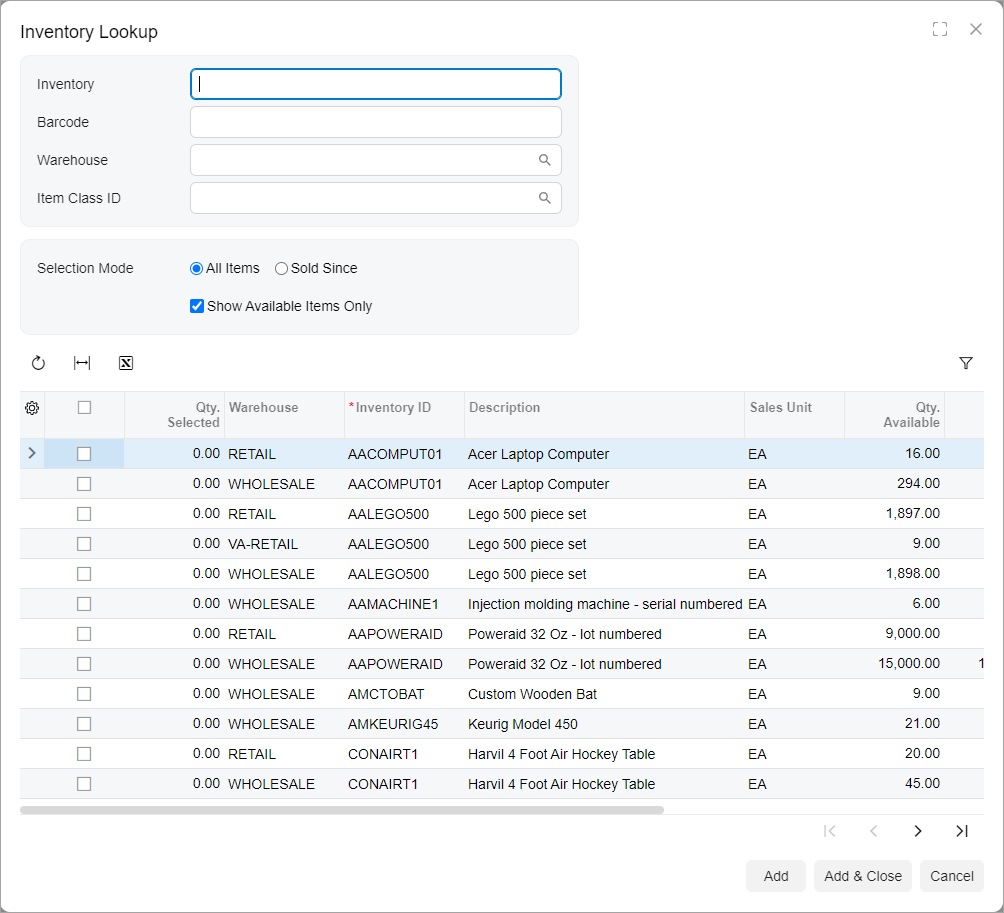
To define the layout of the Summary area of a dialog box, you use one of the predefined templates. For details, see Form Layout: Predefined Templates. The following code shown an example of the HTML for the dialog box in the preceding screenshot.
<qp-panel id="ItemInfo"
caption="Inventory Lookup" auto-repaint="true" width="85vw" height="85vh">
<qp-template name="17-17-14" id="ItemFilter_formSitesStatus"
wg-container class="equal-height">
<qp-fieldset slot="A" id="fs-sitestatusfilter-first" view.bind="ItemFilter">
<field name="Inventory"></field>
<field name="BarCode"></field>
<field name="SiteID"></field>
<field name="ItemClass"></field>
<field name="SubItem"></field>
</qp-fieldset>
<qp-fieldset slot="B" id="fs-sitestatusfilter-second" view.bind="ItemFilter">
<field name="Mode" control-type="qp-radio"></field>
<field name="HistoryDate"></field>
<field name="OnlyAvailable"></field>
<field name="DropShipSales"></field>
</qp-fieldset>
<qp-fieldset slot="C" id="fs-sitestatusfilter-third" view.bind="ItemFilter">
<field name="CustomerLocationID"></field>
</qp-fieldset>
</qp-template>
<qp-grid id="gripSiteStatus" view.bind="ItemInfo" wg-container="ItemInfo_gripSiteStatus">
</qp-grid>
<footer>
<qp-button id="btnAdd" state.bind="AddSelectedItems">
</qp-button>
<qp-button id="btnAddClose" caption="Add & Close" dialog-result="OK">
</qp-button>
<qp-button id="btnCancel" caption="Cancel" dialog-result="Cancel">
</qp-button>
</footer>
</qp-panel>
View Definition of a Dialog Box
The following code shown an example of a view declaration and initialization for a dialog box.
@graphInfo({graphType: 'PX.Objects.SO.SOOrderEntry', primaryView: 'Document',
bpEventsIndicator: true, udfTypeField: 'OrderType', showActivitiesIndicator: true})
export class SO301000 extends PXScreen {
...
AddSelectedItems: PXActionState;
@viewInfo({containerName: "Inventory Lookup"})
ItemFilter = createSingle(SOSiteStatusFilter);
@viewInfo({containerName: "Inventory Lookup"})
ItemInfo = createCollection(SOSiteStatusSelected);
}
export class SOSiteStatusFilter extends PXView {
Inventory: PXFieldState<PXFieldOptions.CommitChanges>;
BarCode: PXFieldState<PXFieldOptions.CommitChanges>;
SiteID: PXFieldState<PXFieldOptions.CommitChanges>;
ItemClass: PXFieldState<PXFieldOptions.CommitChanges>;
SubItem: PXFieldState<PXFieldOptions.CommitChanges>;
Mode: PXFieldState<PXFieldOptions.CommitChanges>;
HistoryDate: PXFieldState<PXFieldOptions.CommitChanges>;
OnlyAvailable: PXFieldState<PXFieldOptions.CommitChanges>;
DropShipSales: PXFieldState<PXFieldOptions.CommitChanges>;
CustomerLocationID: PXFieldState<PXFieldOptions.CommitChanges>;
}
@gridConfig({
preset: GridPreset.Inquiry,
syncPosition: true,
adjustPageSize: true
})
export class SOSiteStatusSelected extends PXView {
@columnConfig({allowCheckAll: true}) Selected: PXFieldState;
QtySelected: PXFieldState;
@columnConfig({hideViewLink: true}) SiteID: PXFieldState;
@columnConfig({hideViewLink: true}) ItemClassID: PXFieldState;
ItemClassDescription: PXFieldState;
@columnConfig({hideViewLink: true}) PriceClassID: PXFieldState;
PriceClassDescription: PXFieldState;
@columnConfig({hideViewLink: true}) PreferredVendorID: PXFieldState;
PreferredVendorDescription: PXFieldState;
@columnConfig({hideViewLink: true}) InventoryCD: PXFieldState;
@columnConfig({hideViewLink: true}) SubItemID: PXFieldState;
Descr: PXFieldState;
@columnConfig({hideViewLink: true}) SalesUnit: PXFieldState;
QtyAvailSale: PXFieldState;
QtyOnHandSale: PXFieldState;
CuryID: PXFieldState;
QtyLastSale: PXFieldState;
CuryUnitPrice: PXFieldState;
LastSalesDate: PXFieldState;
DropShipLastQty: PXFieldState;
DropShipCuryUnitPrice: PXFieldState;
DropShipLastDate: PXFieldState;
AlternateID: PXFieldState;
AlternateType: PXFieldState;
AlternateDescr: PXFieldState;
}