Redirection to Webpages: General Information
You can implement different types of redirection to webpages on an MYOB Acumatica form. For example, you can add a simple redirection link to a form by modifying the ASPX page of the form on which the link is displayed. You can also add conditional logic to the form so that a redirection link opens different forms, depending on whether a specified condition is met. This type of redirection link has to be declared inside an action method.
Learning Objectives
In this chapter, you will learn how to do the following:
- Add a redirection link by using the PXSelector attribute on the ASPX page of a form
- Add a redirection link by implementing an action with a corresponding button on the form
- Redirect the user to a report at the end of the processing operation
Applicable Scenarios
You add redirection to a webpage on a form when you need to give users the ability to open one of the following:
- A record's data entry form with the record displayed, so that a user can get detailed information about that record
- A report or a generic inquiry that is related to the record
- Any destination URL
Redirection Link from a Selector Control
A redirection link from a selector control is often used for opening the data entry form on which the user can edit the record selected in the selector control. You can add redirection from a selector control declaratively by doing the following:
- Adding the PXSelector attribute to a field in its data access class
- Configuring the corresponding element that is generated by default for this field in the ASPX file as a PXSelector element
You set the AllowEdit property of the PXSelector element to true to indicate that the selector should appear as a hyperlink. The DAC that corresponds to the record in the selector control must have the PXPrimaryGraph attribute specified.
Redirection in an Action
To implement redirection in an action, you need to throw one of the exceptions provided by MYOB Acumatica Framework. Once an exception is thrown, it interrupts the current context and propagates up the call stack until it is handled by MYOB Acumatica Framework, which performs the redirection. (This mechanism does not affect the performance of the application.)
The following exceptions are used for redirection:
- PXRedirectRequiredException opens the specified application page in the same window or a new one. By default, the user is redirected in the same window.
- PXPopupRedirectException opens the specified application page in a pop-up window.
- PXReportRequiredException opens the specified report in the same window or a new one. By default, the report opens in the same window.
- PXRedirectWithReportException opens two pages: the specified report in a new window, and the specified application page in the same window.
- PXRedirectToUrlException opens the webpage with the specified external URL in a new window. This exception is also used for opening an inquiry page that is loaded into the same window by default.
The following diagram shows how redirection to a report can be implemented for a processing form.
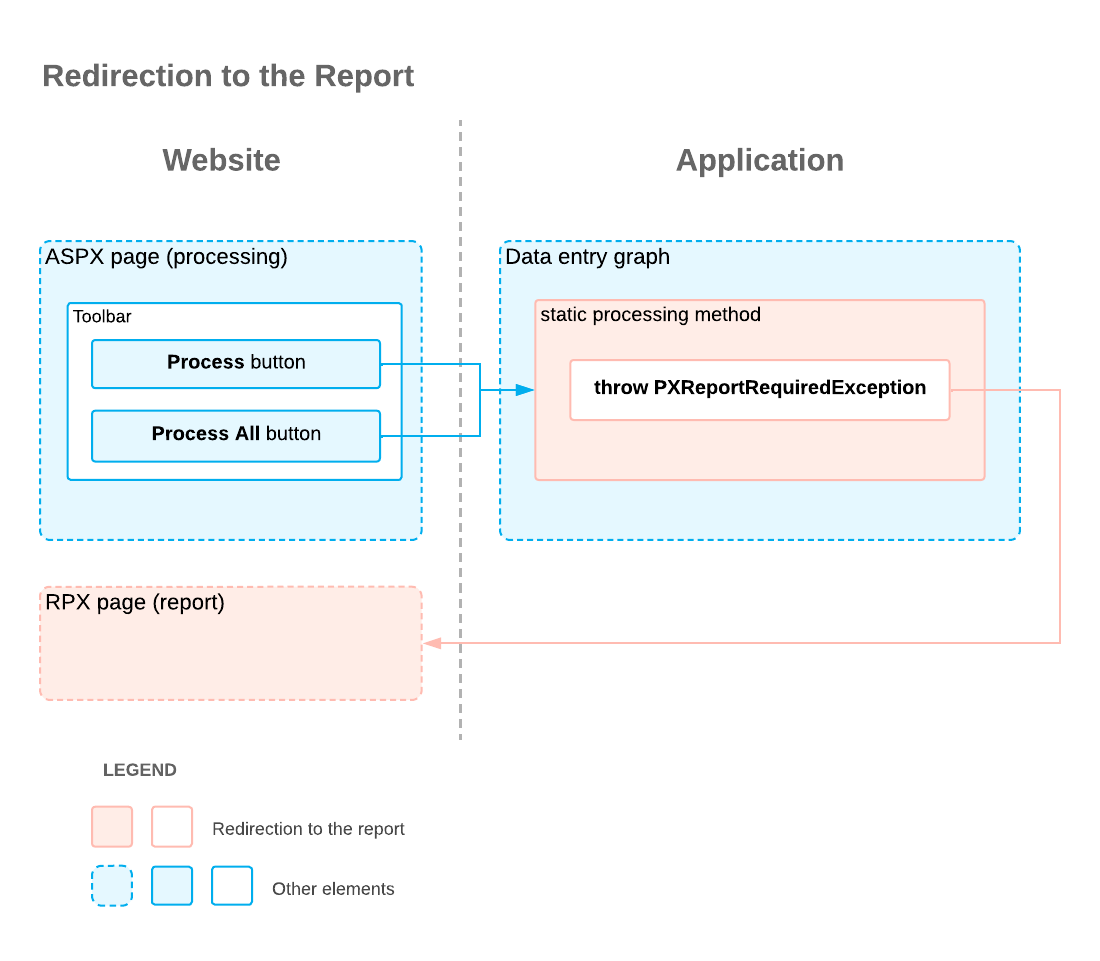
If you want to add to a column a redirection link that is implemented by using an action, you need to specify the action name in the LinkCommand property of the PXGridColumn control.
Handling of Redirection Exceptions
You do not need to implement the handling of the exceptions that are used for redirection in most cases.
catch
block of the exception
handler. The following code example shows how this is
implemented.using PXTransactionScope tranScope = new();
try
{
// Do something that may throw any kind of redirect exception
tranScope.Complete();
}
catch(PXBaseRedirectException redirect){
tranScope.Complete();
throw;
}
Note that in the above code, a PXBaseRedirectException is used. All the exceptions in the preceding list are derived from this base class.