Processing Forms: General Information
On a processing form, a user can invoke an operation on multiple selected records at once. For instance, a processing operation can be a procedure that modifies the status of documents.
Learning Objectives
In this chapter, you will learn how to create a simple processing form (that is, one that does not have any filtering parameters defined).
Applicable Scenarios
You implement a processing form if you need to provide the ability for the user to invoke an operation on multiple records at once.
Processing Forms
Processing forms have a similar appearance to that of inquiry forms. A processing form usually consists of the following components:
- A table that displays the list of records retrieved by the specific processing data
view. The table includes the following components:
- A column with an unlabeled check box, which gives the user the ability to select one record or multiple records in the grid for processing.
- Optional: A redirection button or link that can be clicked to open the data entry form for any selected record.
- A form toolbar that includes the Process, Process All, and Cancel buttons.
- Optional: The area that provides selection criteria (for narrowing the records that are listed and may be processed) or configuration settings (or both) for the processing method.
Naming Conventions for Processing Forms
Processing forms have IDs that start with a two-letter abbreviation (indicating the
functional area of the form) followed by 50 (indicating a processing form), such as
RS501000. The names of the graphs that work with processing forms have the
Process suffix. For instance, RSSVAssignProcess
will be the name
of the graph for the Assign Work Orders (RS501000) form.
For more details about the naming conventions for the ASPX pages and graphs, see Form and Report Numbering and Graph Naming.
Implementation of the Processing Action
Generally, the action that you want to use on a processing form has already been implemented for a data entry form. The action may be implemented as a workflow action or as a graph action. To use this action on a processing form, you need to make the following changes to this action:
- For a graph action, you implement the action handler as follows:
- You move the code from the action handler to the separate static method.
- You change the signature of the action handler so that it returns
IEnumerable
. If you use thevoid
action handler instead, the processing of the long-running operation and its result will not be displayed in the UI. - You replace the PXButton attribute with the PXProcessButton attribute to indicate that the action will be used on the processing form.
- To run the processing method within the action handler, you invoke the PXLongOperation.StartOperation() method, which starts
execution of the processing method in a separate thread. The use of the
PXLongOperation.StartOperation() method is the only way to
execute the processing method asynchronously in MYOB Acumatica Framework. Before you run the operation, you invoke a method to save the last changes made on the data entry form, to be sure to process the latest version of the record.Note: You need to call Save.Press() instead of Actions.PressSave() in an action that is used in a workflow and that starts a long-running operation.
- In the separate static method, you make the following changes to the code of the graph
action:
- You modify the code so that it works with the list of records obtained from the input parameter of the method.
- You add the
isMassProcess
parameter to the method. IfisMassProcess = true
is passed in the method parameters (which means that the method is invoked from a processing form), you can return a successful processing message to the UI by using the static PXProcessing<T>.SetInfo() method. - To handle any errors that might occur during the processing, you enclose the
processing code in the
try
statement. If any error occurs, in thecatch
statement, you use the static PXProcessing<T>.SetError() method to return the processing result for each record to the UI.
- If the action is used in a workflow, you modify the action definition in the workflow so
that the action can be used on the processing form.
In the action definition in the workflow, you call the MassProcessingScreen<>() method with the processing graph as the type parameter. You also call the InBatchMode() method if the workflow action works with the list of records.
You can find details about the implementation of processing actions in Processing Forms: Implementation of Processing Operations.
Definition of the Processing Graph and Data View
- You define the data view for the processing form.To define the data view for the processing form, you use the SelectFrom<Table>.ProcessingView class. This class is derived from the PXProcessingBase<Table> class, which is a base class for the data views of processing forms.Tip: You can also use one of the types that use the traditional BQL style of data queries, such as PXProcessing or PXProcessingJoin.
- You add the
Cancel
action to the processing graph.To define theCancel
action, you use the PXCancel class. If the processing form does not have a filter, you use the main DAC of the processing data view as the type parameter, as shown in the following code.// Definition of the Cancel button for processing without filtering public class SalesOrderProcess : PXGraph<SalesOrderProcess> { public SelectFrom<SalesOrder>.ProcessingView SalesOrders; // Main DAC of the processing data view public PXCancel<SalesOrder> Cancel; }
- You add the processing actions to the processing graph.
By default, any form that has a data view of a type derived from PXProcessingBase<Table> has the Process and Process All buttons on the form toolbar. You can replace the names of the default buttons in the graph constructor. To override the button captions, you use the SetProcessCaption() and SetProcessAllCaption() methods.
- You specify the action to be used for processing.If you use a workflow action for processing, in the RowSelected event handler, you specify the workflow action that the processing form should use for processing by invoking the
SetProcessWorkflowAction<>()
method of the data view.Note: We recommend that you not call theFor the forms that do not use workflow actions for processing, you must specify the processing delegate by using one of theSetProcessWorkflowAction<>()
method in the graph constructor because this could cause incorrect initialization of the workflow.SetProcessDelegate
methods.
Controls for the Processing Form
You add the unbound Selected
data field of the Boolean type to the DAC that
provides the records to process for the processing form and then add the column for this
field to the form. If a user doesn’t want to process all listed records, the user will
select the check box in this column for each record to be processed. You define the
Selected
data field as unbound by using the PXBool
type attribute. (Unlike the PXDBBool attribute, the
PXBool attribute does not have the DB part in its name. The
presence of the DB part indicates a bound data type.)
Selected
is the default name for the data field for this specific check
box; you can define the data field with any name and override the default
Selected
name in the graph constructor with the
SetSelected() method of the PXProcessing
class.You make all columns in the grid (except for the column that corresponds to the
Selected
field) unavailable for editing by specifying
SkinID="Inquire"
for the grid. For the Selected
column,
you set the AllowCheckAll property of the corresponding control to
True
to make it possible for the users to select all records listed on
the current page of the table for processing by selecting the check box in the column
header.
Implementation Summary and Diagram
For a simple processing form that displays data to be processed and provides processing actions, you usually define the following:
- In the processing graph, the specific SelectFrom<Table>.ProcessingView (derived from PXProcessingBase) data view type to provide data records for the form
- In the graph constructor, the names of the processing buttons
- In the RowSelected event handler, the workflow action to be used for processing
- In the DAC, the unbound
Selected
data field, which is used to indicate the records to be processed - In the ASPX page, the column in the grid for the
Selected
data field
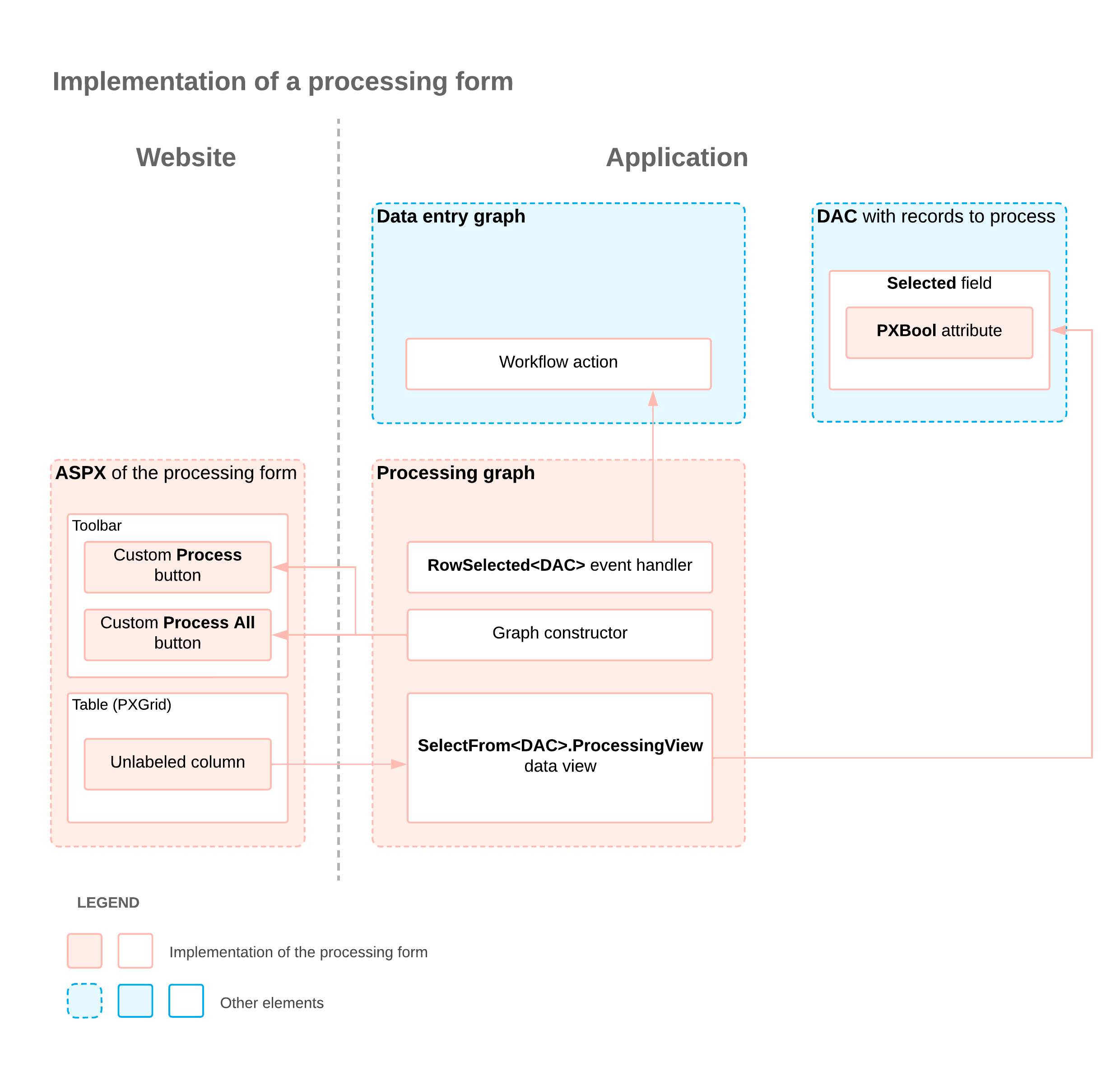