Filtering Parameters: General Information
When a user specifies selection criteria on a form, the system displays in the table the data narrowed by the selection criteria. On an inquiry or processing form, the use of selection criteria gives users the ability to view the most relevant data. On a processing form, users can then process all of the currently listed records or only those they select.
For both of these types of forms, you can define filtering parameters to give users the ability to filter the data listed in the table.
Learning Objectives
In this chapter, you will learn how to add filtering parameters to a form.
Applicable Scenarios
You add filtering parameters in the following cases:
- For a processing form, you need to provide the ability for the user to filter the records before processing.
- For an inquiry form, you want to give users the ability to view data narrowed down by custom filtering parameters, and reusable filters are not sufficient to provide the needed functionality.
DAC with Filtering Parameters
- The DAC contains the fields that correspond to the filtering parameters.
- The DAC contains only unbound fields because you do not read the values of the parameters from the database.
- The DAC does not contain any key fields because the DAC works with only one data record that represents the current filtering parameters.
You usually assign the PXHidden attribute to the filter DAC because you do not need this DAC to be used in generic inquiries and reports.
To be able to use the filtering parameters in a BQL query, you need to make sure that the fields defined in this DAC are added to the grid view DAC of the form.
Filter Data View
The generic PXFilter type of data view is used to provide filtering parameters for user selection on an MYOB Acumatica form, such as an inquiry form or a processing form. The PXFilter data view always creates a single data record and never retrieves this data record or saves it to the database. The PXFilter data view is used to specify values that are used by the application logic or other data views and that never should be stored anywhere except the current user session.
The PXFilter data view always returns one record with the current values of the filtering parameters. The PXFilter data view works only with the UI and does not invoke any requests to the database. The data view object for the filtering parameters is defined in a graph, as the following code shows.
public class RSSVPaymentPlanInq : PXGraph<RSSVPaymentPlanInq>
{
//RSSVWorkOrderToAssignFilter is a DAC with filtering parameters
public PXFilter<RSSVWorkOrderToAssignFilter> Filter;
...
}
Other Graph Members
To display the filtered data, the graph must contain the data view that selects the records that meet the criteria specified by the filtering parameters. For details about this data view, see Filtering Parameters: Filtered Data on a Processing Form and Filtering Parameters: Filtered Data on an Inquiry Form.
To clear the filtering parameters on the form, you define the Cancel
action
(which actually clears all data on the form) for the filter DAC; see the following code
example.
public PXFilter<RSSVWorkOrderToAssignFilter> Filter;
// Adds the form toolbar button that clears the filtering parameters
public PXCancel<RSSVWorkOrderToAssignFilter> Cancel;
You should also override the IsDirty
property of the graph to make the
IsDirty
property always return false. This disables the dialog box
that confirms that a user wants to leave the form. This dialog box appears when a user
attempts to close the form if there are unsaved changes in the cache objects for the form. A
false value in the IsDirty property of the graph means that
there are no unsaved changes on the form and that the dialog box never appears. This
behavior makes sense on processing and inquiry forms, which are not intended for data entry
or editing.
You can also use the PXUIFieldAttribute.SetEnabled<>() method in the graph constructor to enable editing for particular data fields.
Changes to the ASPX Page
Filter data fields are usually displayed on a form. To immediately refresh data records as soon a user updates a filtering parameter, enable callback for the input control that displays the filtering parameter on the form (see To Enable Callback for a Control).
The PXFilter data view is specified in the PrimaryView property of the datasource control of the ASPX page where the filtering parameters are used, as shown in the following code example.
<px:PXDataSource ID="ds" ...
PrimaryView="Filter" TypeName="PhoneRepairShop.RSSVPaymentPlanInq">
Implementation Summary and Diagram
To add a filter to a form, you generally complete the following steps:
- You define the DAC that provides the filtering parameters.
- You modify the DAC that provides records for filtering by adding the fields that correspond to the filtering parameters.
- In the graph, you define the following members:
- The
Cancel
action - The data view of the PXFilter type, which provides data for the filter
- The data view that retrieves filtered records
For details about this data view, see Filtering Parameters: Filtered Data on a Processing Form or Filtering Parameters: Filtered Data on an Inquiry Form.
- The
- In the graph, you modify the following members:
- The graph constructor: To enable editing of particular columns in the table with the filtered results
- The
IsDirty
property: To disable the dialog box that confirms that a user wants to leave the form
- In the ASPX page, you add the PXFormView container with the elements that correspond to the filtering parameters.
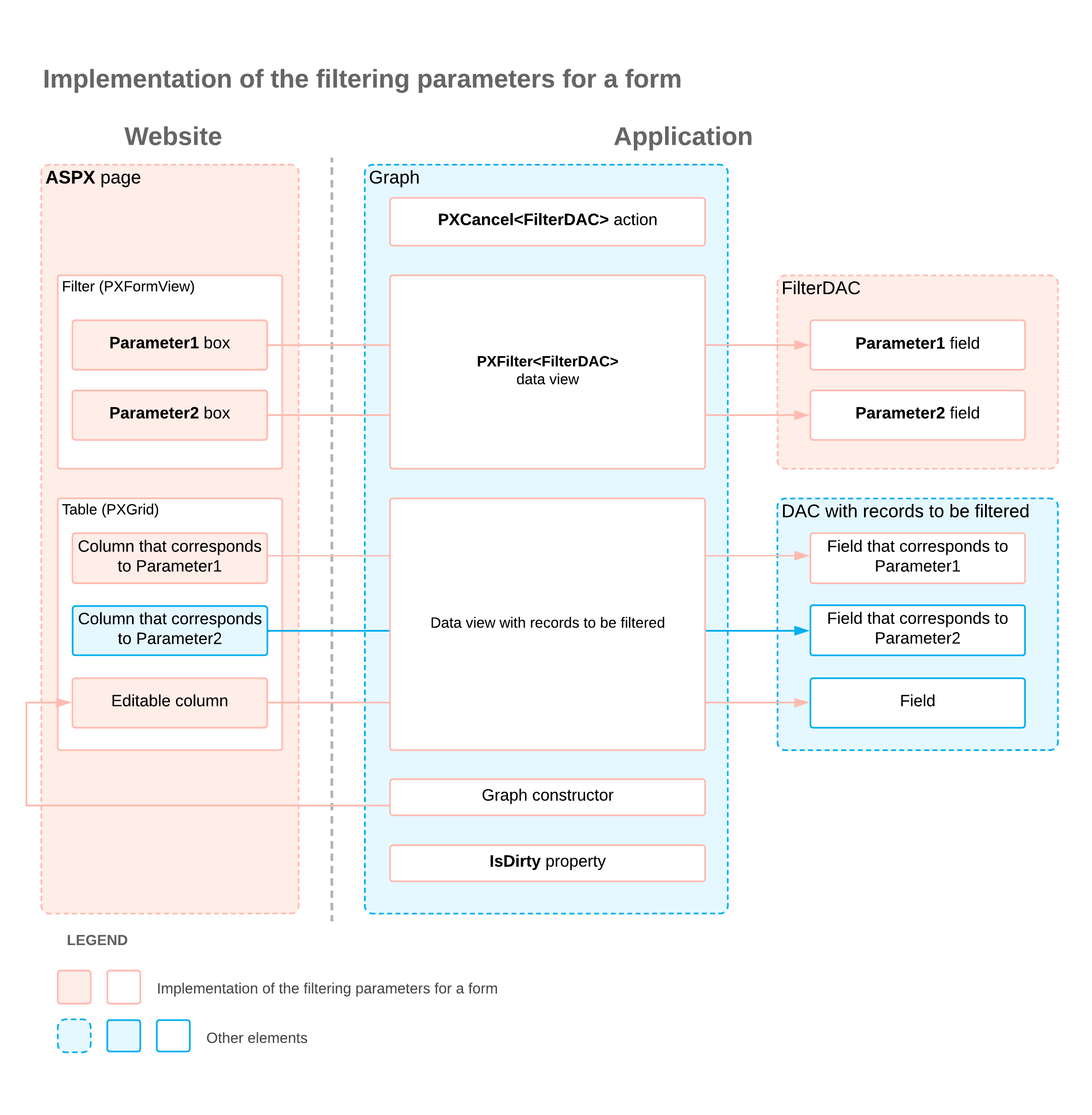