Configuration of Selector Controls
You use selector controls to provide a list from which the user can select a data record and then to set the ID of the selected data record as the data field value.
Defining a Selector Control
To configure a selector control, you use the PXSelector attribute in the definition of the data field in the data access class (DAC), as shown in bold type in the following example.
[PXDBInt(IsKey = true)]
[PXDefault]
[PXUIField(DisplayName = "Product ID")]
[PXSelector(typeof(Search<Product.productID>),
typeof(Product.productCD),
typeof(Product.productName),
typeof(Product.unitPrice),
SubstituteKey = typeof(Product.productCD))]
public virtual int? ProductID
...
In the first parameter, you specify a Search<> BQL query to select data records for the control. The Search<> command has the same syntax as the Select<> command, except that you specify the data field of the main DAC. In the Search<> command, you can specify conditions and join data from other DACs. When a user selects a data record in the control, the control assigns the value of the specified field to the data field.
WHERE
, JOIN
, ORDER
BY
, or GROUP BY
conditions. Thus, in the example above, you can
specify typeof(Product.productID)
instead of
typeof(Search<Product.productID>)
in the first parameter.Defining the List of Columns
You configure the columns that should be shown in the control by providing the types of the fields after the Search<> command; see the code in bold type in the following example.
[PXSelector(typeof(Search<Product.productID>),
typeof(Product.productCD),
typeof(Product.productName),
typeof(Product.unitPrice),
SubstituteKey = typeof(Product.productCD))]
public virtual int? ProductID
...
The code above defines three columns (see the following screenshot).
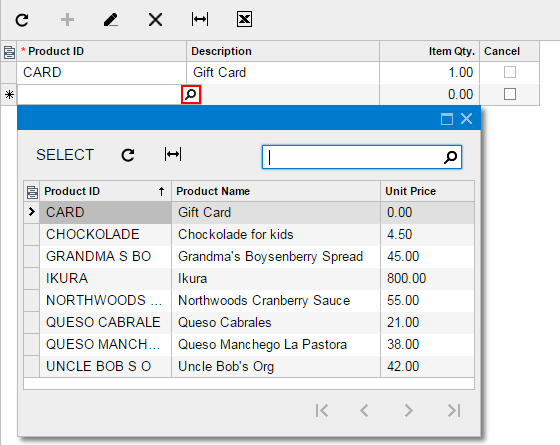
You can join multiple DACs in the Search<> command and specify fields
from the joined DACs as columns. The following code example shows in bold type the
LeftJoin
clause and the fields from the joined DAC added to the selector
control as columns.
[PXDBString(10, IsKey = true, IsUnicode = true, InputMask = "")]
[PXDefault]
[PXUIField(DisplayName = "Shipment Nbr.")]
[PXSelector(typeof(
Search2<Shipment.shipmentNbr,
LeftJoin<Customer, On<Customer.customerID,
Equal<Shipment.customerID>>>>),
typeof(Shipment.shipmentNbr),
typeof(Shipment.customerID),
typeof(Customer.customerCD),
typeof(Customer.companyName))]
public virtual string ShipmentNbr
...
Replacing the Displayed Key Value
The SubstituteKey property specifies the field whose value should be shown in the control in the UI instead of the field that is specified in the Search<> command.
The SubstituteKey property is shown in the bold type in the following code.
[PXSelector(typeof(Search<Product.productID>),
typeof(Product.productCD),
typeof(Product.productName),
typeof(Product.minAvailQty),
SubstituteKey = typeof(Product.productCD))]
public virtual int? ProductID
...
In the example above, the ProductID field of a shipment line stores the ProductID value of the selected product, while in the UI. the control shows the ProductCD value. Conversion between the ProductID and ProductCD values happens in the FieldUpdating and FieldSelecting event handlers, which are implemented within the PXSelector attribute.