Barcode Scan Transitions: General Information
ScanTransition<TScanBasis> is a class for a barcode scan transition. This class represents a component that defines the rules of automatic transitions between scan states.
Learning Objectives
- Implement transitions between scan states
- Implement a simple transition flow between scan states
Applicable Scenarios
You implement scan transitions in the following cases:
- You have defined a set of scan states of a custom scan mode. You need to implement transitions between these states.
- You have defined a new scan state of a predefined scan mode. You need to implement transitions between the predefined scan states and the new scan state.
Transition Between Scan States
- Call Basis.SetScanState(string state) or Basis.SetScanState<TScanState>() to change the current scan state to another certain scan state. This way requires a particular state to be specified and is not flexible because scan states become highly coupled.
- Call
Basis.DispatchNext()
to indicate to the system that the current scan state should be moved further. This way does not include information about a specific next scan state. The system derives the information about the next scan state from the transition map, which is defined by the scan transition component.
Transition Map
You can use the scan transition component to create a complex map of transitions by using conditions and additional actions that can be performed when the transition is triggered.
Unlike all other scan components, ScanTransition<TScanBasis> is not
an abstract
class. However, it still can be configured by a condition or by
additional transition logic. Also, in some cases, a transition map does not contain any
branches; it contains only a group of optional states. In these cases, the creation of the
transition map can be simplified by the ScanStateFlow<TScanBasis>
class, which you can use to create the transition map sequentially.
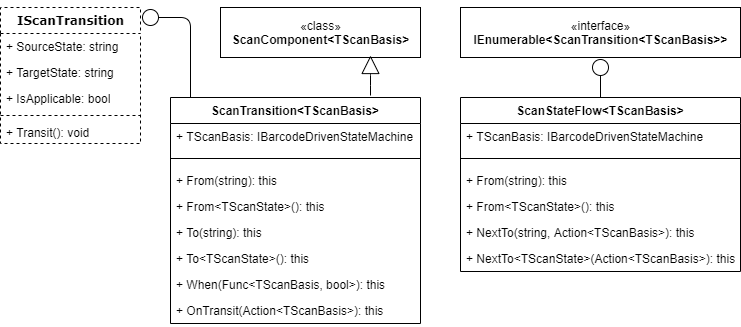
Implementation of the Transition Map
You create particular instances of the ScanTransition<TScanBasis> class in the ScanMode<TScanBasis>.CreateTransitions() method.
To define the transition map, you can use the following methods:
- Transition(Func<ScanTransition<TSelf>, ScanTransition<TSelf>>
config)
The Transition object can be used for producing a branching structure of transitions. This approach is shown in Barcode Scan Transitions: To Implement Transitions.
- StateFlow(Func<ScanStateFlow<TSelf>.IFrom, ScanStateFlow<TSelf>.IFlow>
config)
The StateFlow object implements the IEnumerable<ScanTransition<TScanBasis>> interface—that is, it produces a sequence of transition objects. The StateFlow object cannot be used for producing a branching structure of transitions. However, it supports additional actions that can be performed upon transition. To specify this actions, you use the overload of the NextTo method that accepts an Action<TScanBasis> delegate. This approach is shown in Barcode Scan Transitions: To Implement a Simple Transition Flow.